COMPUTER PROGRAMMING , IOE ,TU
1.3 Problem-Solving using a Computer
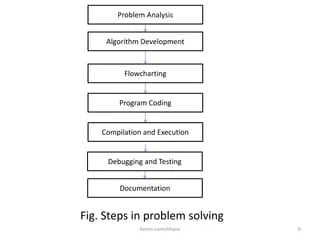
1.3.1 Problem Analysis
If you have studied a problem statement, then you must analyze the problem and determine how to solve it. First, you should know the type of problem, that is, the nature of the problem. From a programming point of view, the problem must be computing. At first you try to solve it manually. If it is solvable manually by using your idea and knowledge, then you can use such ideas and principles in programming and solve the problem by using a computer. So, you must have good knowledge about a problem. In order to get an exact solution, you must analyze the problem. To analyze means you should try to know the steps that lead you to have an exact solution.
1.3.2 Algorithm
An algorithm is a step by step description of activities or methods to be processed for getting desired output from a given input. OR
An algorithm is the step by step description of the procedure written in human understandable language for solving given problems.
Example
- Write an algorithm to find the sum of two numbers.
Step 1: Start
Step 2: Declare variables num1, num2 and sum.
Step 3: Read values for num1, num2.
Step 4: Add num1 and num2 and assign the result to a variable sum.
Step 5: Display sum
Step 6: Stop
|
Properties
- Finiteness: Program should have a finite number of steps to solve a problem.
- Definiteness: The action of each step should be defined clearly without any ambiguity.
- Inputs: Inputs of the algorithms should be defined precisely, which can be given initially or while the algorithm runs.
- Outputs: Each algorithm must result in one or more outputs.
- Effectiveness: It should be more effective among the different ways of solving the problems.
Guidelines for writing algorithm
- Use plain language. In any example, the English language is used.
- Do not use any programming language specific syntax.
- Every job to be done should be described clearly without any assumptions.
- The developed algorithm must have a single entry and exit point.
1.3.3.Flowchart
A flowchart is a pictorial representation of an algorithm that uses boxes of different shapes to denote different types of instructions. The actual instructions are written within these boxes using clear and concise statements. These boxes are connected by solid lines having arrow marks to indicate the flow of operation, that is, the exact sequence in which the instructions are to be executed.
Advantages of Using Flowcharts
- Communication: Flowcharts are a better way of communicating the logic of a system to all concerned or involved.
- Effective analysis: With the help of flowchart, problems can be analyzed in a more effective way therefore reducing cost and wastage of time.
- Proper documentation: Program flowcharts serve as a good program documentation, which is needed for various purposes, making things more efficient.
- Efficient Coding: The flowcharts act as a guide or blueprint during the systems analysis and program development phase.
- Proper Debugging: The flowchart helps in the debugging process.
- Efficient Program Maintenance: The maintenance of operating program becomes easy with the help of flowchart. It helps the programmer to put efforts more efficiently on that part
Disadvantages of Using Flowcharts:
- Complex logic: Sometimes, the program logic is quite complicated. In that case, flowchart becomes complex and clumsy. This will become a pain for the user, resulting in a waste of time and money trying to correct the problem.
- Alterations and Modifications: If alterations are required the flowchart may require re- drawing completely. This will usually waste valuable time.
- Reproduction: As the flowchart symbols cannot be typed, reproduction of flowchart becomes a problem.
Flowchart Symbols
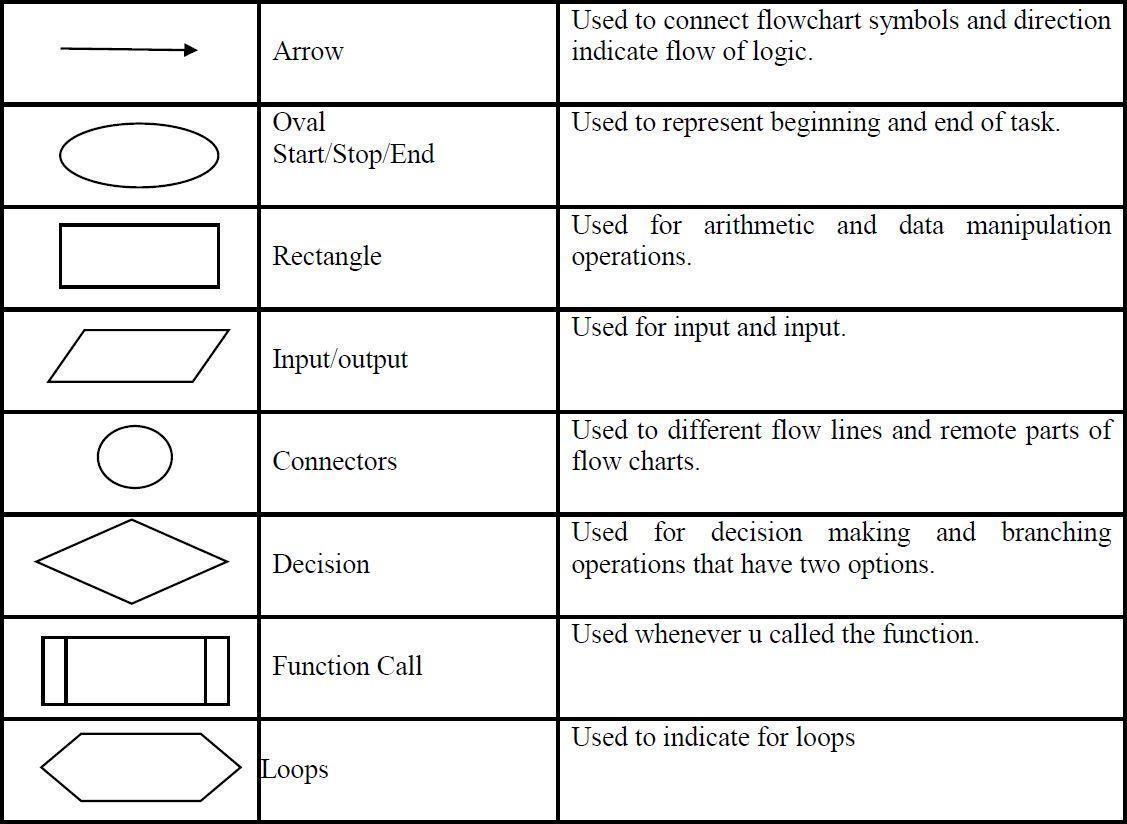
Guidelines to be followed to draw a flowchart
- The flowchart should be clear, neat and easy to follow.
- The usual direction of the flow of a procedure or system is from left to right or top to bottom.
- Only one flow line should come out from a process symbol.
- Only one flow line should enter a decision symbol, but two or three flow lines, one for each possible answer, should leave the decision symbol.
- If the flowchart becomes complex, it is better to use connector symbols to reduce the number of flow lines. Avoid the intersection of flow lines if you want to make it a more effective and better way of communication.
- Ensure that the flowchart has a logical start and finish.
- It is useful to test the validity of the flowchart by passing through it with a simple test data
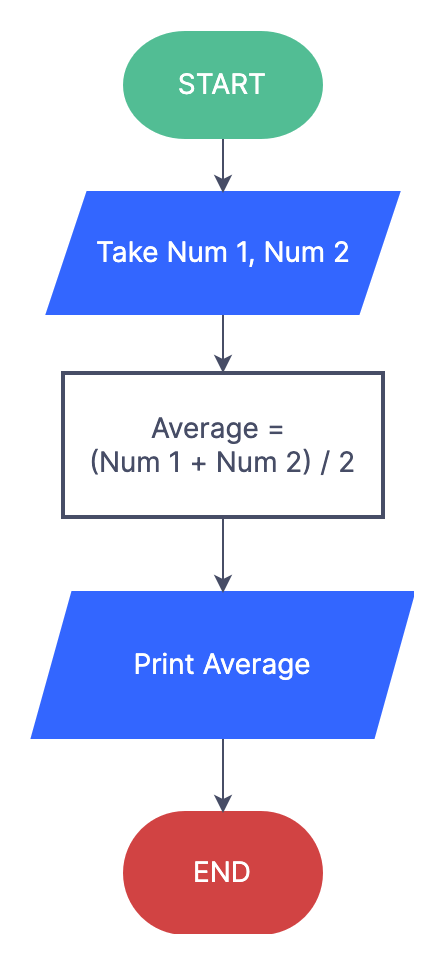
1.3.4 Programming (Coding)
In order to make a program in any programming language, what we have written is known as code. The act of writing code in a computer language is known as coding. In other words, code is a set of instructions that a computer can understand.
1.3.5 Compilation, Linking and Execution
The process by which source codes of a computer (programming) language are translated into machine codes is known as compilation. After compilation if everything is ok, the code is going under another process that is known as execution. We can get the required output after the execution.
Compiler:
The job of a compiler includes the following:
- To translate High level language source programs to machine codes.
- To trace variables in the program.
- To allocate memory for storage of programs and variables.
- To generate error messages, if there are errors in the program.
Compilation Process
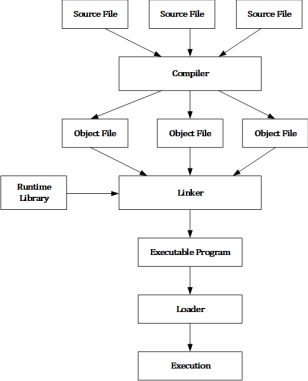
Figure: Illustration of compilation process
The process of translation from high level language (source code) to low level language (object code) is called compilation.
- The first step is to pass the source code through a compiler, which translates the high level language instructions into object code.
- The final step in producing an executable program is to pass the object code through a linker. The linker combines modules and gives real values to all symbolic addresses, thereby producing machine code.
- Compilation process ends producing an executable program.
- The compiler stores the object and executable files in secondary storage.
- If there is any illegal instruction in the source code, the compiler lists all the errors during compilation.
Interpreter
The basic purpose of an interpreter is the same as that of a compiler. In the compiler, the program is translated completely and a directly executable version is generated. Whereas interpreter translates each instruction, executes it and then the next instruction is translated and this goes on until end of the program. In this case, object code is not stored and reused. Every time the program is executed, the interpreter translates each instruction freshly. It also has program diagnostic capabilities. However, it has some disadvantages as below:
- Instructions repeated in the program must be translated each time they are executed.
- Because the source program is translated fresh every time it is used, it is a slow process or execution takes more time. Approx. 20 times slower than compiler.
- Interpretation means the process by which high level language is translated into machine level language by interpreter and program is translated and executed line by line.
Assembler:
Assembler is a computer program which is used to translate a program written in Assembly Language into machine language. The translated program is called an object program. Assembler checks each instruction for its correctness and generates diagnostic messages, if there are mistakes in the program. Various steps of assembling are:
- Input source program in Assembly Language through an input device.
- Use Assembler to produce object programs in machine language.
- Execute the program.
Difference between compiler and Interpreter:
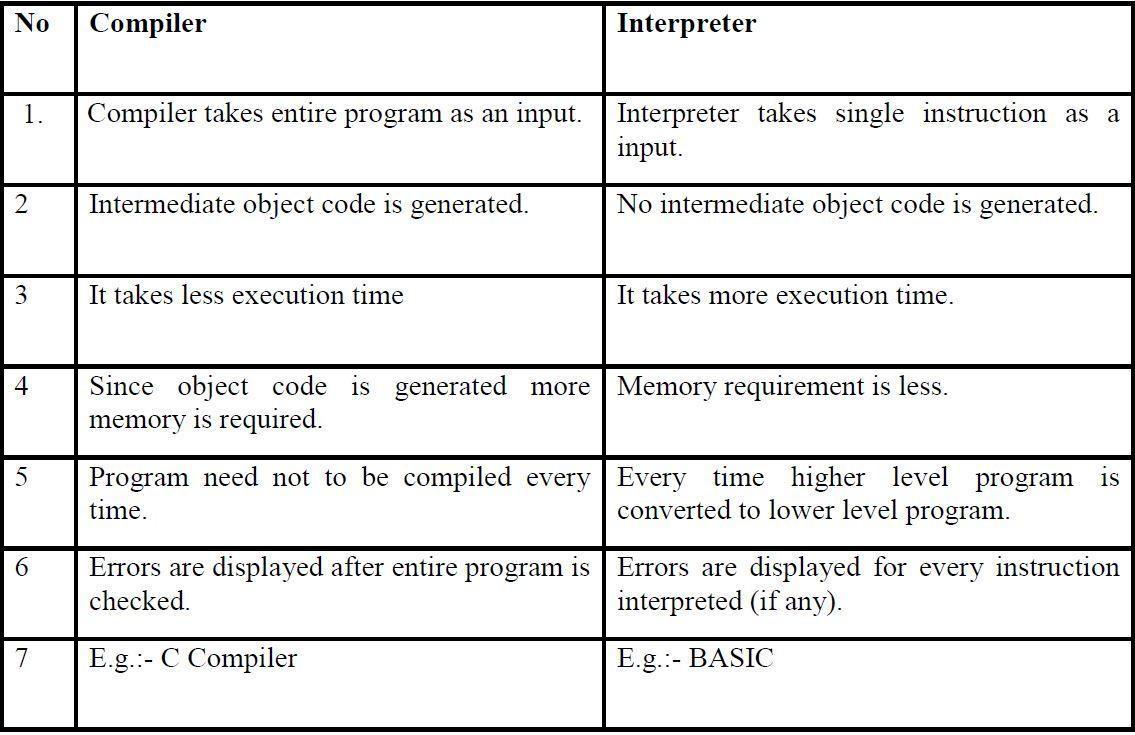
Difference between compiler and assembler:
COMPILER
|
ASSEMBLER
|
Generates the assembly language code or directly the executable code.
|
Generates the relocatable machine code.
|
Preprocessed source code.
|
Assembly language code.
|
The compilation phases are lexical analyzer, syntax analyzer, semantic analyzer,intermediate code generation, code optimization, code generation.
|
Assembler makes two passes over the given input.
|
The assembly code generated by the compiler is a mnemonic version of machine code.
|
The relocatable machine code generated by an assembler is represented by binary code.
|
1.3.6 Debugging and Testing
It is the process of detecting and removing errors in a program, so that the program produces the desired results on all occasions. Debugging is the discovery and correction of programming errors. Testing ensures that the program performs correctly the required task. Before going into details about the debugging and testing process, different errors that can occur should be discussed.
Errors
While writing C programs, errors also known as bugs in the world of programming may occur unwillingly which may prevent the program to compile and run correctly as per the expectations of the programmer.
Basically 4 different types:
1.Runtime Errors
Runtime errors are those errors that occur during the execution of a c program and generally occur due to some illegal operations that were performed in the program.
Examples of some illegal operations that may produce runtime errors are:
- Dividing a number by zero.
- Trying to open a file which is not created.
- Lack of free memory space.
It should be noted that occurrence of these errors may stop program execution, thus to encounter this, a program should be written such that it is able to handle such unexpected errors and rather than terminating unexpectedly, it should be able to continue operating. This ability of the program is known as robustness and the code used to make a program robust is known as guard code as it guards program from terminating abruptly due to occurrence of execution errors.
2.Compile Errors
Compile errors are those errors that occur at the time of compilation of the program. C compile errors may be further classified as:
- Syntax Error
- When the rules of the c programming languages are not followed, the compiler will show syntax errors.For example, consider the statements, int a,b:
- The above statement will produce syntax error as the statement is terminated with : rather;
- Semantic Errors
- Semantic errors are reported by the compiler when the statements written in the c program are not meaningful to the compiler.For example, consider the statement, b+c=a;
- In the above statement, we are trying to assign value of a in the value obtained by summation of b and c which has no meaning in c. the correct statement will be
- a=b+c;
- Logical Errors
- Logical errors are the errors in the output of the program. The presence of logical errors leads to undesired or incorrect output and are caused due to error in the logic applied in the program to produce the desired output. Example: Using > sign when less than < was required.
- Also, logical errors could not be detected by the compiler, and thus, programmers has to check the entire coding of a c program line by line.
- Latent Errors
- The hidden errors that shows up only when a particular set of data is used. Example: r=(x+y)/(p-q)
- This expression shows up error when p=q.
Debugging
It is the process of isolating and correcting different type of errors. Different debugging techniques are given below.
- Error Isolation
- It is used for locating an error resulting in a diagnostic message.
- We can generally find the location of errors by temporarily deleting a certain portions of program code and rerunning the program to see whether the error is again appeared or not.
- If error is not appeared then , deleted portion of programs has error. And if error again appears then that deleted portion is error free.
- Here in this process, temporary deletion is done by commenting those portion of program.
- Tracing
- In this technique, printf() statement is used to print the values of some important variables at different stages of program.
- If any value is incorrect, we can easily fix the location of the error.
- Watch Values
- A watch value is the value of variable or an expression, which is displayed continuously as the program executes.
- Thus we can see the changes in a watch value as they occur, in response to the program logic.
- By inspecting these values carefully, we can determine where the program begins to generate an incorrect or unexpected value.
- Breakpoints
- It is a temporary stopping point with in a program.
- Each breakpoint is associated with a particular instruction within the program.
- When the program is executed, the program execution will temporarily stop at the breakpoint before the instruction is executed.
- Execution may be resumed until next breakpoint is encountered.
- Breakpoints are often used in conjunction with watch values.
- Debug Add Breakpoints provide the requested information in the dialog box. Or



- Select a particular line within the program press function F5. To disable the breakpoint again press function key F5.

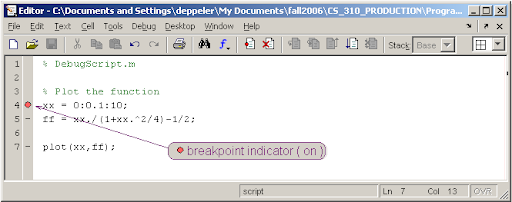
- Stepping
- The process of executing one instruction at a time is called execution.
- We can determine which instruction produce erroneous result or generate error message by stepping through the entire program.
- Stepping is often used with watch values, allowing us to trace the entire history of a program as it executes.
Testing
It is the process of executing a program or system with the intent of finding errors. It involves any activity aimed at evaluating an attribute or capability of a program or system and determining that it meets its required results. Testing is usually performed for the following purposes:
- To improve quality.
- For verification and validation.
- For reliability estimation.
Testing process may include the following two stages:
Human Testing
- It is an effective error detection process and is done before the computer based testing begins.
- It includes code inspection by the programmer and test group and review by a peer group.
- The test is carried out statement by statement and is analyzed with respect to checklist of common programming errors.
- In addition to finding the errors, the programming style and choice of algorithm are also reviewed.
Computer Based Testing
- It involves two stages namely compile testing and run time testing.
- Compile testing can show different syntax errors and run time errors may produce the run time error message such as null pointer assignment and stack overflow.
- After removing errors, it is required to run the program with test data to check whether the program is producing the correct result or not.
- Program testing can be done either at module (function) level i.e. unit testing or program level .
- An integration testing is done to find the errors associated with interfacing.
Black Box Testing
It is a software testing method in which the internal structure/ design/ implementation of the item being tested is not known to the tester.
White Box Testing
It is a software testing method in which the internal structure/ design/ implementation of the item being tested is known to the tester.
Difference between Testing and Debugging
Testing
|
Debugging
|
The purpose of testing is to find bugs and errors.
|
The purpose of debugging is to correct those bugs found during testing.
|
Testing is done by tester.
|
Debugging is done by programmer or developer.
|
It can be automated.
|
It can’t be automated.
|
It can be done by outsider like client.
|
It must be done only by insider i.e. programmer.
|
Most of the testing can be done without design knowledge.
|
Debugging can’t be done without proper design knowledge.
|
1.3.7 Documentation
Program Documentation refers to the details that describe a program. While writing programs, it is good programming practice to make a brief explanatory note on the programmer program segment. This explanatory note is called a comment. It explains how the program works and interacts with it. Thus, it helps other programmers to understand the program. Program documentation is used for both the original programmer and beginner. The final document should contain the following information:
- A program analysis document with objectives, inputs, outputs and processing procedures.
- Program design document algorithm and detailed flowchart and other appropriate diagrams.
- Program verification documents, with details of checking, testing and correction procedures along with the list of test data.
- Log is used to document future program revision and maintenance activity.